Output Formatting
We have seen the basic ability to convert values to strings and output them to the console ([[stdout]]). Sometimes, however, we would like more control on the formatting used to output values. Fortunately we have formatted output functions that help us with that goal.
C-Style printf()
In the olden days when the C language was developed in the 1970's it contained a novel new way of formatting output called the printf()
function. This function allows one to specify a format string followed by values that will be substituted into the format string based on the type specifiers found in the format string. This approach has proven very popular and has been copied in many languages since.
With formatted output we must provide a format string that can contain literal text interspersed with type specifiers. The type specifiers are placeholders that identify where and how a value is to be formatted and inserted into the string on output. Type specifiers start with a percent sign (%
), followed by an optional flag, followed by field width and/or precision, followed by one or two characters identifying the type of the value (called the type specifier). Only the percent sign and the type specifier is required, the other components are optional.
%[flag][width][.precision][type specifier]
In addition to the format string, we also provide the values to be formatted to the function. We can have multiple type specifiers in the format string as long as we provide the matching number of additional arguments. The first argument is formatted by the first type specifier, the second argument by the second type specifier, and so on.
In JavaScript the console.log()
method supports a limited set of printf()
style specifiers. For a full implementation see the sprintf()
discussion later on this page.

Any other characters in the format string that are not part of a type specifier are printed verbatim. For example:
console.log("Page count is %d", numPages); console.log("PI: %f", pi); console.log("Title and Page Count: %s %d", title, numPages); console.log("%d of %d", currentPage, numPages);
Some of the type specifiers available are listed in the table below.
Specifier | Value Type | Description |
---|---|---|
d or i | integral | Formatted number |
f | floating point | Formatted number |
j | Object | JSON formatted object |
o | Object | Clickable object |
s | String | Formatted string of object |
JavaScript console.log()
does not support the flags, width or precision fields of a print specifier. See sprintf()
section below for a JavaScript function that does support the full range of printf()
style print specifiers.
#!/usr/bin/env node; const main = async () => { const a = 326; const b = -1; const c = 2015; const i1 = 65000; const i2 = -2; const i3 = 3261963; const f1 = 3.1415926; const f2 = 2.99792458e9; const f3 = 1.234e-4; const c1 = "A".codePointAt(0); const c2 = "B".codePointAt(0); const c3 = "C".codePointAt(0); const s1 = "Apples"; const s2 = "and"; const s3 = "Bananas"; const b1 = true; const b2 = false; console.log("Decimals: %d %d %d", a, b, c); console.log("Long Decimals: %d %d %d", i1, i2, i3); console.log("Fixed FP: %f %f %f", f1, f2, f3); console.log("Boolean: %d %d", b1, b2); console.log("Boolean: %s %s", b1, b2); console.log("Character: %s %s %s", String.fromCodePoint(c1), String.fromCodePoint(c2), String.fromCodePoint(c3)); console.log("String: %s %s %s", s1, s2, s3); } main().catch( e => { console.error(e) } );
Output
C-Style sprintf()
Along with the printf()
function, the C language introduced a similar function called sprintf()
. Instead of printing a formatted string to the console ([[stdout]]), it formats and then returns a string. This more general form has many uses not only for console output but for [[GUI]] output and file output.
sprintf()
for JavaScript from [[sprintf-js]]. If using node.js it can be installed using the Node Package Manager on the command line.
Specifier | Value Type | Description |
---|---|---|
b | integer | Binary |
d or i | integer | Signed decimal |
u | integer | Unsigned decimal |
x | integer | Hexadecimal (lowercase) |
X | integer | Hexadecimal (uppercase) |
f | floating point | Fixed notation |
e | floating point | Scientific notation (lowercase) |
E | floating point | Scientific notation (uppercase) |
g | floating point | Shortest representation: %e or %f |
G | floating point | Shortest representation: %E or %F |
c | integer | Single character |
s | string | String |
t | boolean | True or False |
T | any | Yields the type |
v | any | Yields the primitive value |
% | none | Outputs literal % |
Flag | Description |
---|---|
- | Left-justify within the given field width; Right justification is the default. |
+ | Result is preceeded with a plus or minus sign (+ or -) even for positive numbers. By default, only negative numbers are preceded with a - sign. |
# | Used with o, x or X specifiers the value is preceeded with 0, 0x or 0X respectively. Used with e, E, f, g or G it forces the written output to contain a decimal point even if no more digits follow. By default, if no digits follow, no decimal point is written. |
0 | Left-pads the number with zeroes (0) instead of spaces |
#!/usr/bin/env node; const Utils = require('./Utils'); const sprintf = require('sprintf-js').sprintf; const main = async () => { const a = 326; const b = -1; const c = 2015; const i1 = 65000; const i2 = -2; const i3 = 3261963; const f1 = 3.1415926; const f2 = 2.99792458e9; const f3 = 1.234e-4; const c1 = "A".codePointAt(0); const c2 = "B".codePointAt(0); const c3 = "C".codePointAt(0); const s1 = "Apples"; const s2 = "and"; const s3 = "Bananas"; const b1 = true; const b2 = false; let s; s = sprintf("Decimals: %d %d %d", a, b, c); console.log(s); s = sprintf("Long Decimals: %d %d %d", i1, i2, i3); console.log(s); s = sprintf("Fixed FP: %f %f %f", f1, f2, f3); console.log(s); s = sprintf("Boolean: %t %t", b1, b2); console.log(s); s = sprintf("Boolean: %s %s", b1, b2); console.log(s); s = sprintf("Character: %s %s %s", String.fromCodePoint(c1), String.fromCodePoint(c2), String.fromCodePoint(c3)); console.log(s); s = sprintf("String: %s %s %s", s1, s2, s3); console.log(s); } main().catch( e => { console.error(e) } );
Output
String Interpolation
Many languages have the ability to replace variables written directly into strings. This variable substitution in strings is know as variable interpolation.
In JavaScript we have template literals that implement the idea of variable interpolation. Template literals are strings enclosed in backticks (`
) instead of single or double quotes like normal strings. We use the syntax ${variable_name}
to represent the place in the string where we want the value of our variable to be replaced. In addition to simple variable names, we can also place simple expressions inside the curly quotes of a interpolation placeholder.
#!/usr/bin/env node; const main = async () => { const FICA_RATE = 0.0765; const PAY_RATE = 20.; let annual_salary; annual_salary = PAY_RATE * 2080.0; console.log(`FICA Tax Rate: ${FICA_RATE}`); console.log(`Annual Salary: ${annual_salary}`); console.log(`FICA Tax: ${FICA_RATE * annual_salary}`); } main().catch( e => { console.error(e) } );
Output
Modern Message Formatting
The methods for formatting strings and output discuss so far have some limitations when it comes to localizing software. The positional approaches taken by the printf()
style functions poses difficulties to localization because often during translation the order of words and thus the substition specifiers must change but the hard-code argument list in our code can not change to match. Variable interpolation has its drawbacks because you are actually putting code into the strings. When externalizing the strings (removing them from the code and putting them in a separate file) necessary for localization, it is not desirable to export variables and expressions from our code to the localization file where they can be changed.
This is why message formatting approaches have been developed that use substitution specifiers that specify which argument to the message format function is to be used. This allows the substitution specifiers to be in a different order (and perhaps re-ordered during localization) than the formal arguments to the message format function. These substitution specifiers are also not executable code as is the case with variable interpolation so it is much safer to externalize from our program code as we will see in the section on Internationalization.
A format()
function that is modelled after the Python format()
function is provided in the [[Pure Programmer JavaScript Utils Module]]. Save the "Utils.js" source in the same folder where your source files reside. Once this is done, you may use the require for "./Utils" to be able to use the Utils module in your programs. It does require the sprintf-js
module to be installed in order to work for floating point values.
#!/usr/bin/env node var Utils = require('./Utils'); var first = "First"; var middle = "Middle"; var last = "Last"; var s = Utils.format("{2}, {0} {1}", first, middle, last); console.log(s);
The format()
method uses a pair of curly brackets to identify substition placeholders in the format string. Each pair of curly brackets contains a number from 0 to the number of addtional arguments - 1. This number refers to the position in the argument list of the value that will be used in the substitution. This allows values in the argument list to be used in any order needed in the format string or even used more than once.
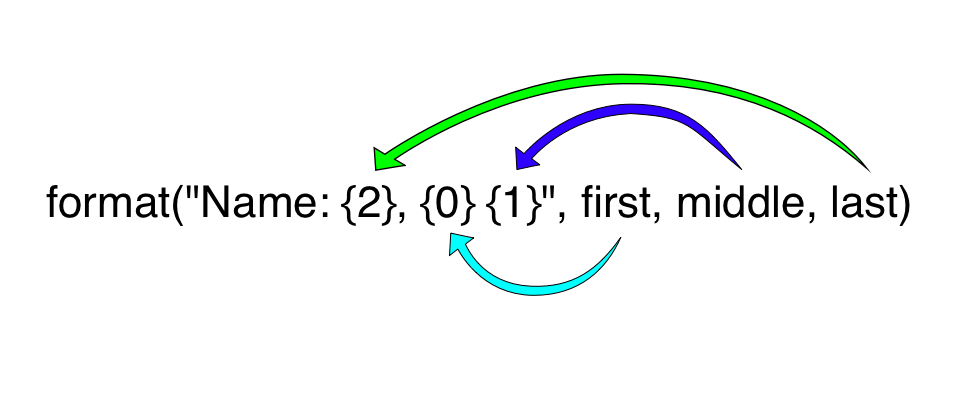
Following the position number in the substitution placeholder is an optional type specifier. The type specifier is separated from the position number by a colon. If you don't wish to use a type specifier then the type is assumed to be string. Some of the type specifiers available are listed in the table below.
Specifier | Value Type | Description |
---|---|---|
b | integer | binary |
d | integer | decimal |
o | integer | octal |
x | integer | hexadecimal (lowercase) |
X | integer | hexadecimal (uppercase) |
f | double | fixed notation |
e | double | scientific notation (lowercase) |
E | double | scientific notation (uppercase) |
g | double | shortest representation: e or f |
G | double | shortest representation: E or f |
c | integer | single character |
s | string | string of characters |
Like we have seen in printf()
, the format()
specifiers can take optional modifiers that change how the value is to be formatted. The full definition of the specifiers, including optional components is as follows:
{[arg-pos]:[fill-and-align][sign][#][0][width][.precision][type specifier]}
The fill-and-align option is an optional fill character (which can be any character other than {
or }
), followed by one of the align options <
, >
, ^
. If no fill character is specified, then the space character is used.
Option | Description |
---|---|
< | Left-justify within the given field width. This is the default for non-numeric types. |
> | Right-justify within the given field width. This is the default for numeric types. |
^ | Aligns the value in the center of the field. |
The sign option is one of +
, -
or the space character.
Option | Description |
---|---|
+ | Signifies that a sign character should be output for all values (+ for positive and - for negative). |
- | Signifies that a sign character should be output for negative values only. This is the default for numeric types. |
<space> | Signifies that a leading space should be used for positive values and a - should be output for negative values.
|
The #
option causes alternate formatting to be used.
- For integral types, when binary, octal, or hexadecimal presentation type is used, the alternate form inserts the prefix (0b, 0, or 0x) into the output value after the sign character.
- For floating-point types, the alternate form causes the result of the format to always contain a decimal-point character, even if no digits follow it.
The 0
option pads the field with leading zeros (following any indication of sign or base) to the field width. If the 0
option and an align option both are used, the 0 option is ignored.
The width option is a positive decimal number. If present, it specifies the minimum field width. If the formatted value can not fit within the field width the entire value will be inserted causing the field to be larger than width.
The precision option is a .
followed by a non-negative decimal number. This option indicates the precision to use when formatting a value. For floating-point types, precision specifies the formatting precision, i.e. the number of places after the decimal point to display. For string types, it specifies how many characters from the string will be used.
#!/usr/bin/env node; const Utils = require('./Utils'); const main = async () => { const first = "First"; const middle = "Middle"; const lastname = "Last"; const left = "Left"; const center = "Center"; const right = "Right"; const favorite = "kerfuffle"; const i1 = 3261963; const i2 = -42; const fp1 = 3.1415926; const fp2 = 2.99792458e9; const fp3 = -1.234e-4; let s = Utils.format("{2}, {0} {1}", first, middle, lastname); console.log(s); s = Utils.format("{0} {1} {2}", left, center, right); console.log(s); s = Utils.format("Favorite number is {0}", i1); console.log(s); s = Utils.format("Favorite FP is {0}", fp1); console.log(s); let c = Utils.cpAt(favorite, 0); console.log(Utils.format("Favorite c is {0:c}", c)); console.log(Utils.format("Favorite 11c is |{0:11c}|", c)); console.log(Utils.format("Favorite <11c is |{0:<11c}|", c)); console.log(Utils.format("Favorite ^11c is |{0:^11c}|", c)); console.log(Utils.format("Favorite >11c is |{0:>11c}|", c)); console.log(Utils.format("Favorite .<11c is |{0:.<11c}|", c)); console.log(Utils.format("Favorite _^11c is |{0:_^11c}|", c)); console.log(Utils.format("Favorite >11c is |{0: >11c}|", c)); c = 0x1F92F; console.log(Utils.format("Favorite emoji c is {0:c}", c)); console.log(Utils.format("Favorite s is {0:s}", favorite)); console.log(Utils.format("Favorite .2s is {0:.2s}", favorite)); console.log(Utils.format("Favorite 11s is |{0:11s}|", favorite)); console.log(Utils.format("Favorite 11.2s is |{0:11.2s}|", favorite)); console.log(Utils.format("Favorite <11.2s is |{0:<11.2s}|", favorite)); console.log(Utils.format("Favorite ^11.2s is |{0:^11.2s}|", favorite)); console.log(Utils.format("Favorite >11.2s is |{0:>11.2s}|", favorite)); console.log(Utils.format("Favorite .<11.2s is |{0:.<11.2s}|", favorite)); console.log(Utils.format("Favorite *^11.2s is |{0:*^11.2s}|", favorite)); console.log(Utils.format("Favorite ->11.2s is |{0:->11.2s}|", favorite)); console.log(Utils.format("Favorite d is {0:d}", i1)); console.log(Utils.format("Another d is {0:d}", i2)); console.log(Utils.format("Favorite b is {0:b}", i1)); console.log(Utils.format("Another B is {0:b}", i2)); console.log(Utils.format("Favorite o is {0:o}", i1)); console.log(Utils.format("Another o is {0:o}", i2)); console.log(Utils.format("Favorite x is {0:x}", i1)); console.log(Utils.format("Another X is {0:X}", i2)); console.log(Utils.format("Favorite #b is {0:#b}", i1)); console.log(Utils.format("Another #B is {0:#b}", i2)); console.log(Utils.format("Favorite #o is {0:#o}", i1)); console.log(Utils.format("Another #o is {0:#o}", i2)); console.log(Utils.format("Favorite #x is {0:#x}", i1)); console.log(Utils.format("Another #X is {0:#X}", i2)); console.log(Utils.format("Favorite 11d is |{0:11d}|", i1)); console.log(Utils.format("Favorite +11d is |{0:+11d}|", i1)); console.log(Utils.format("Favorite 011d is |{0:011d}|", i1)); console.log(Utils.format("Favorite 011x is |{0:011x}|", i1)); console.log(Utils.format("Favorite #011x is |{0:#011x}|", i1)); console.log(Utils.format("Favorite f is {0:f}", fp1)); console.log(Utils.format("Another f is {0:f}", fp2)); console.log(Utils.format("One more f is {0:f}", fp3)); console.log(Utils.format("Favorite e is {0:e}", fp1)); console.log(Utils.format("Another e is {0:e}", fp2)); console.log(Utils.format("One more e is {0:e}", fp3)); console.log(Utils.format("Favorite g is {0:g}", fp1)); console.log(Utils.format("Another g is {0:g}", fp2)); console.log(Utils.format("One more g is {0:g}", fp3)); console.log(Utils.format("Favorite .2f is {0:.2f}", fp1)); console.log(Utils.format("Another .2f is {0:.2f}", fp2)); console.log(Utils.format("One more .2f is {0:.2f}", fp3)); console.log(Utils.format("Favorite .2e is {0:.2e}", fp1)); console.log(Utils.format("Another .2e is {0:.2e}", fp2)); console.log(Utils.format("One more .2e is {0:.2e}", fp3)); console.log(Utils.format("Favorite .2g is {0:.2g}", fp1)); console.log(Utils.format("Another .2g is {0:.2g}", fp2)); console.log(Utils.format("One more .2g is {0:.2g}", fp3)); console.log(Utils.format("Favorite 15.2f is |{0:15.2f}|", fp1)); console.log(Utils.format("Another 15.2e is |{0:15.2e}|", fp2)); console.log(Utils.format("One more 15.2g is |{0:15.2g}|", fp3)); } main().catch( e => { console.error(e) } );
Output
Questions
- What is the difference in output between the printf() specifier "%8d" and "%8x"?
- What is the difference in output between the printf() specifier "%8d" and "%-8d"?
- What is the difference in output between the printf() specifier "%8d" and "%+8d"?
- What is the difference in output between the printf() specifier "%f" and "%e"?
- What is the difference in output between the printf() specifier "%f" and "%g"?
- How many characters from the string are printed when using "%10.8s"?
- How many characters characters in total are printed when using "%10.8s"?
- When using "%5.3f" how is 34.5678 formatted?
- When using "%.1f%%" how is 12.345 formatted?
- What is the difference in output between the format() specifier {0:010x} and {0:+10x}?
- What is the difference in output between the format() specifier {0:+5.2f} and {0:-5.2f}?
- What is the difference in output between the format() specifier {0:^20s} and {0:<20s}
Projects
More ★'s indicate higher difficulty level.
- Aligning Characters and Strings with format()
- Aligning Characters and Strings with printf()
- Aligning Floating Point with format()
- Aligning Floating Point with printf()
- Aligning Integers with format()
- Aligning Integers with printf()
- Combined FICA Tax (Formatted)
References
- [[sprintf-js]]