Getting Started with Javascript
Every programming language has some boilerplate material that we use to start a programming project. Mostly it is code to describe where the program should start and what additional libraries should be used to augment the programming language itself. Thus we have, in every language, this smallest program that we can write. We will ignore some of the explanation as to exactly what these minimal programs are doing until later. It is sufficient for now that we treat this as boilerplate code that forms the foundation of our programs. We can then expand upon these boilerplate programs as we explore the various features of the language.
JavaScript is unique among the languages that we explore on this site because JavaScript programs can be run as stand-alone programs or as embedded programs within a web page. When writing web-based applications JavaScript (along with C++, Java, Perl and Python) can be used to write the server-side of the web application. Unlike these other languages, JavaScript is the only language that we can use to write the client-side because there is a JavaScript interpreter embedded in every popular web browser. To run JavaScript code in a web browser, we will create an HTML file that contains embedded JavaScript.
<!DOCTYPE html> <html lang="en"> <head> <title>Getting Started</title> </head> <body> <script type="text/javascript"> document.writeln("Hello, world!"); </script> </body>
When running as a stand-alone program we can write pure JavaScript files. Below is an example JavaScript program that can be written usine the [[node.js]] interpreter.
#!/usr/bin/env node; /****************************************************************************** * Simple first program. * * Copyright © 2020 Richard Lesh. All rights reserved. *****************************************************************************/ const main = async () => { console.log("Hello, world!"); } main().catch( e => { console.error(e) } );
Output
This approach to introducing a programming language by writing a simple program that outputs one phrase, typically "Hello, world!" was first seen in Brian Kernighan and Dennis Ritchie's book "The C Programming Language" in the 1970s.
Language Execution Models
In order to get our programs as they are written in our favorite language to run on a computer, it is necessary to convert from the English-like text of our source code to something that the computer CPU can understand. Depending on the language, this either involves a compilation or interpretation phase. Some modern languages require both phases.
Compilation involves taking our source code and then translating it into another language, typically the language of the CPU known as machine language. Compilation is typically performed by a program called a compiler and it is a required step when writing in a compiled language. Once our program has been compiled into machine code, we can then run it directly on the CPU. Languages such as C, C++, Objective C, Swift, FORTRAN and Cobol are all compiled languages. These languages are typically used in the development of operating systems and commercially distributed code.
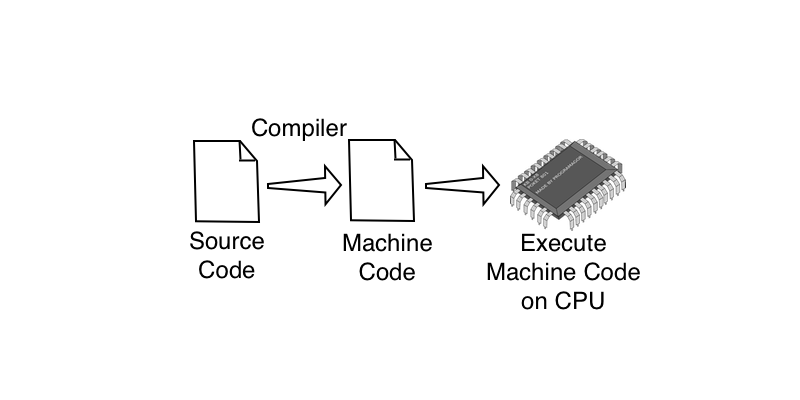
Interpretation involves taking our source code and then line by line decomposing the source into steps that are immediately carried out by the CPU. Interpreted languages require a program called an interpreter to perform this function. There is no compilation phase, we just get to the business of running our program straight from the source code each time we want it to run. Compared to compiled languages, it can often be easier to develop and debug interpreted programs. Interpreted languages, often called scripting languages, include JavaScript, Python and Ruby.
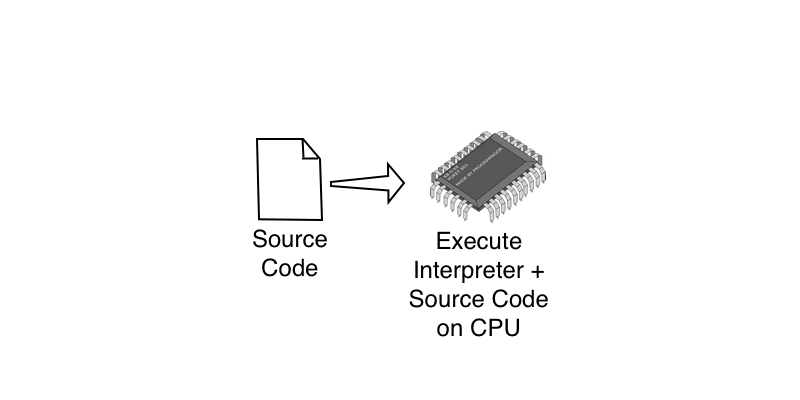
Some languages, most notable are Java and JavaScript, use both approaches. In the case of Java, the source code is compiled to the machine code for a fictional CPU known as the virtual machine (VM). Because your CPU doesn't typically use the same instruction set as the virtual machine, the compiled program must then be interpreted by a program that in essence pretends to be the virtual machine. So languages that use a VM have both a compiled and an interpreted phase. Notable VM languages are Java and C#.

Tool Setup
Because JavaScript uses the Interpreted Language Execution Model we need to have the following software tools available to us: programming text editor to create/edit our source files, interpreter software to run our source files, and a command-line console to launch the program and handle the input and output from our program.
Command-Line Console
The Command-Line Console is used to invoke the JavaScript interpreter and is also used by the programs we write for text input and output. Linux, Mac OS X and Windows all come with a Command-Line Console program though many are not accustomed to using it.
- In Linux the Command-Line Console is immediately available if booting in text mode. If booting in the Gnome graphical interface, it is typically available under the Application Menu -> Accessories -> Terminal.
- In Mac OS X the Command-Line Console is found in the Applications folder -> Utilities -> Terminal.
- On the Raspberry Pi the Command-Line Console is immediately available if booting in text mode. If booting in the Gnome graphical interface, it is available under the Application Menu -> Accessories -> Terminal.
- In Windows the Command-Line Console is found under the Start Menu -> All Programs -> Accessories -> Command Prompt. On Windows there are many more functional terminal emulator programs than the built-in one available such [[ConsoleZ]].
On this site we will use the terms console, terminal and command-line prompt interchangeably. Illustrations of terminal command sequences will be presented in a box with a black background. User input will appear in white while the command prompts ($) and program output will appear in green.
$ user input program output
Programming Text Editor
Programming Text Editors can be as simple as a plain text editor or as complicated as a text editor built-in to an Integrated Development Environment (IDE). For our purposes a middle-of-the-road programming editor will suffice. These types of text editors offer some niceties beyond the basic ability to edit text files. The most common of these programming oriented features is syntax highlighting which colors the parts of the programming text to indicate its role in the programming language's syntax. Other features include auto-completion, auto-balancing of brackets and auto-indenting of source code.
Programming Text Editors are not the same types of programs as Word Processing software. Word Processing software is designed to format and layout complex text documents such as manuals, brochures or flyers. The documents that they create are stored in special word processing formats that are not compatible with our programming tools. Programming Text Editors not only have features aimed at helping write programs, but more importantly they save the source files in a plain text format ([[ASCII]] or [[UTF-8]]) required by our programming tools.
While bare-bones text editors that come with your OS like TextEdit (Mac OS X), LeafEdit (Raspbian) or Notepad (Windows) will work to edit our source code files, you will be more productive using a programming text editor. Based on your operating system you might try some of the programming text editors listed below.
- Linux: [[GEdit]] (Free), [[Geany]] (Free), [[Komodo Edit]] (Free), [[Sublime Text]] (Free), [[UltraEdit]], [[Visual Studio Code]] (Free)
- Mac OS X: [[GEdit]] (Free), [[Geany]] (Free), [[BBEdit]], [[Komodo Edit]] (Free), [[Sublime Text]] (Free), [[TextMate 2]], [[UltraEdit]], [[Visual Studio Code]] (Free)
- Raspberry Pi: [[GEdit]] (Free), [[Geany]] (Free), [[Visual Studio Code]] (Free)
- Windows: [[GEdit]] (Free), [[Geany]] (Free) [[Komodo Edit]] (Free), [[Notepad++]] (Free), [[Sublime Text]] (Free), [[UltraEdit]], [[Visual Studio Code]] (Free)
Most Linux distributions based on the Gnome desktop will come with GEdit already installed. Some, however, like the distribution for the Raspberry Pi do not. If your distribution does not have GEdit installed it is easy to install from the command-line terminal using apt
.
$ sudo apt install gedit
Raspberry Pi OS comes with Geany already installed. If your Linux distribution does not have Geany installed it is easy to install from the command-line terminal using apt
.
$ sudo apt install geany
Visual Studio Code can be installed on Linux distributions including Raspberry Pi OS from the command-line terminal using apt
.
$ sudo apt install code
Interpreter
Since JavaScript interpreters are part of most modern web browsers, make sure that you have one or more of the following web browsers installed. If you click on the download icon of the example at the top of this page, you should see a blank page with the text "Hello, world!" displayed. If you do not, then either your browser does not have JavaScript or it does and it has been disabled. Look in your browsers security settings and make sure JavaScript has not been turned off.
- [[Chrome]], [[Chromium]], [[Firefox]], [[Midori]], [[Opera]], [[Web/Epiphany]]
- [[Chrome]], [[Edge]], [[Firefox]], [[Opera]], [[Safari]]
- [[Chromium]], [[Midori]], [[Web/Epiphany]]
- [[Chrome]], [[Edge]], [[Firefox]], [[Midori]], [[Opera]], [[Web/Epiphany]]
If you would rather explore JavaScript using stand-alone programs instead of web browser hosted programs, you can use the [[node.js]] environment. Installers for node.js can be downloaded from the node.js website. Linux distributions can install node.js using apt
.
$ sudo apt install nodejs npm
To see if your system has the node.js interpreter tool chain installed, use the terminal to print the version of your installed interpreter.
The node.js interpretor for Windows can be downloaded from the node.js website. Once installed you can run it from the Windows command prompt or the [[Cygwin]] terminal.
References
- [[JavaScript Language Reference]], Mozilla Developer Network
- [[Mozilla Developer Network]]
- Download [[node.js]]
- [[w3schools.com]]